Free Trial
Web API version
Licensing
Request A Quote
HAVE QUESTIONS OR NEED HELP? SUBMIT THE SUPPORT REQUEST FORM or write email to SUPPORT@BYTESCOUT.COM
Decode EAN-13 | C#
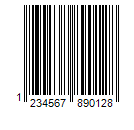
Program.cs:
C#
using System; using System.IO; using Bytescout.BarCodeReader; namespace ReadEAN13 { class Program { const string ImageFile = "EAN13.png"; static void Main() { Console.WriteLine("Reading barcode(s) from image {0}", Path.GetFullPath(ImageFile)); Reader reader = new Reader(); reader.RegistrationName = "demo"; reader.RegistrationKey = "demo"; // Set barcode type to find reader.BarcodeTypesToFind.EAN13 = true; /* ----------------------------------------------------------------------- NOTE: We can read barcodes from specific page to increase performance. For sample please refer to "Decoding barcodes from PDF by pages" program. ----------------------------------------------------------------------- */ // Read barcodes FoundBarcode[] barcodes = reader.ReadFrom(ImageFile); foreach (FoundBarcode barcode in barcodes) { Console.WriteLine("Found barcode with type '{0}' and value '{1}'", barcode.Type, barcode.Value); } // Cleanup reader.Dispose(); Console.WriteLine("Press any key to exit.."); Console.ReadKey(); } } }